mdbox
Just simple markdown utils
Just simple markdown utils!
💡 Why?
Markdown is intended to be as easy-to-read and easy-to-write as is. Readability is emphasized above all else. A Markdown-formatted document should be publishable as-is, as plain text ^1. Any sequence of characters is a valid Markdown document ^2.
While Markdown is designed to be simple, I often find myself in situations where there is simply no tool to allow programmatically working with Markdown syntax without dealing with complex and strict AST objects and choosing between dozens of available tools and extensions. Often, not even worth pursuing ideas around Markdown.
The idea is to make good-enough tools to read and write Markdown programmatically, as easy as Markdown itself is, without dealing with an AST.
Usage
Install package:
# npm
npm install mdbox
# yarn
yarn add mdbox
# pnpm
pnpm install mdbox
# bun
bun install mdbox
Import:
// ESM
import { md } from "mdbox";
// CommonJS
const { md } = require("mdbox");
Render Utils
blockquote(text)
Render a markdown blockquote text with > in front of a paragraph
Example:
md.blockquote("Hello, World!");
// => "> Hello, World!"
bold(text)
Render a markdown bold text.
Example:
md.bold("Hello, World!");
// => "**Hello, World!**"
boldAndItalic(text)
Render a markdown bold and italic text.
Example:
md.boldAndItalic("Hello, World!");
// => "***Hello, World!***"
codeBlock(code, lang?, opts?: { ext? })
Format a string as a code block.
Example:
md.codeBlock('console.log("Hello, World!");', "js");
// => "```js\nconsole.log("Hello, World!");\n```"
heading(text, level)
Render a markdown heading.
Example:
md.heading("Hello, World!", 1);
// => "\n# Hello, World!\n"
hr(length)
Render a markdown horizontal rule.
Example:
md.hr();
// => "---"
image(url, text?, opts?: { title? })
Render a markdown image.
Example:
md.image("https://cataas.com/cat", "Cute Cat");
// => "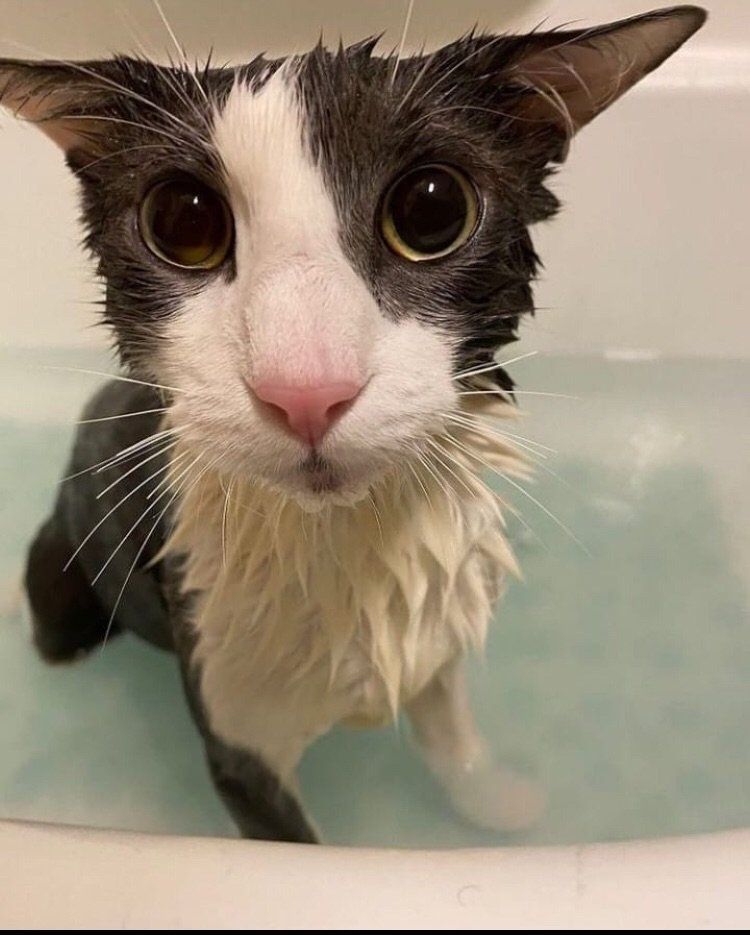"
italic(text)
Render a markdown italic text.
Example:
md.italic("Hello, World!");
// => "_Hello, World!_"
link(url, text?, opts?: { title?, external? })
Render a markdown link.
Example:
md.link("https://www.google.com", "Google");
// => "[Google](https://www.google.com)"
md.link("https://www.google.com", "Google", { external: true });
// => "<a href="https://www.google.com" title="Google" target="_blank">Google</a>"
list(items, opts: { ordered?, char? })
Render a markdown ordered or unordered list.
Example:
md.list(["Item 1", "Item 2", "Item 3"]);
// => "- Item 1\n- Item 2\n- Item 3"
md.list(["Item 1", "Item 2", "Item 3"], { ordered: true });
// => "1. Item 1\n2. Item 2\n3. Item 3"
strikethrough(text)
Render a markdown strikethrough text.
Example:
md.strikethrough("Hello, World!");
// => "~~Hello, World!~~"
table(table: { rows[][], columns[] })
Render a markdown table.
Example:
md.table({
columns: ["Breed", "Origin", "Size", "Temperament"],
rows: [
["Abyssinian", "Egypt", "Medium", "Active"],
["Aegean", "Greece", "Medium", "Active"],
["American Bobtail", "United States", "Medium", "Active"],
["Applehead Siamese", "Thailand", "Medium", "Active"],
],
});
Parsing Utils
initMarkdownItParser(options)
Create parser with markdown-it. WARNING: The returned tree structure is unstable.
Example:
import { initMarkdownItParser } from "mdbox/parser";
const parser = await initMarkdownItParser();
const { tree } = parser.parse("# Hello, *world*!");
initMd4wParser(opts)
Create parser with md4w. WARNING: The returned tree structure is unstable.
Example:
import { initMd4wParser } from "mdbox/parser";
const parser = await initMd4wParser();
const { tree } = parser.parse("# Hello, *world*!");
initMdAstParser(opts)
Create parser with mdast-util-from-markdown. WARNING: The returned tree structure is unstable.
Example:
import { initMdAstParser } from "mdbox/parser";
const parser = await initMdAstParser();
const { tree } = parser.parse("# Hello, *world*!");
Contribution
Local development
License
Published under the MIT license. Made by @pi0 and community 💛